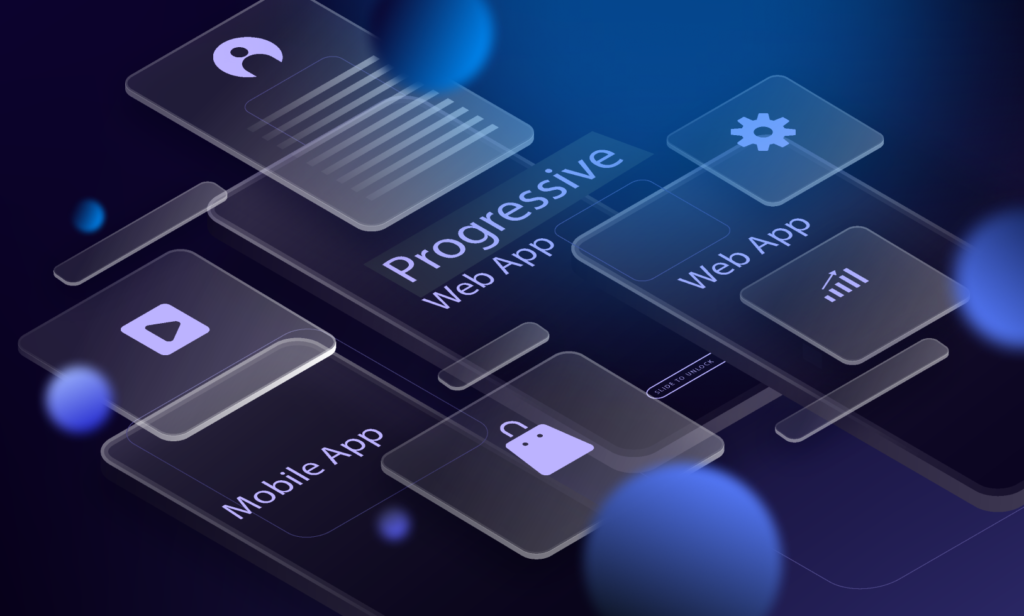
Introduction
Progressive Web Apps (PWAs) are a significant advancement in web development, offering the performance and user experience of native apps while being accessible via the web. For full-stack developers, understanding PWAs is crucial, as they bridge the gap between traditional web applications and native mobile apps. This guide will walk you through what PWAs are, their key features, and how to build and optimise them in the sequence covered in a standard java full stack developer course.
What are Progressive Web Apps (PWAs)?
Progressive Web Apps are web applications that use modern web capabilities to deliver an app-like experience to users. They are built using standard web technologies such as HTML, CSS, and JavaScript but include additional features like offline support, push notifications, and device hardware access to enhance the user experience.
Key Characteristics of PWAs
The key characteristics of PWAs that will be detailed in most full stack developer classes are listed here.
- Progressive: PWAs work for every user, regardless of browser choice, because they are built with progressive enhancement as a core principle.
- Responsive: They fit any form factor, whether a desktop, mobile device, tablet, or something in between.
- Connectivity Independent: PWAs can work offline or on low-quality networks, thanks to service workers that cache essential resources and enable offline functionality.
- App-like Interactions: PWAs mimic the behaviour and feel of native apps, including navigation, user interactions, and UI elements.
- Fresh: They are always up-to-date because of the service worker update process.
- Safe: PWAs are served over HTTPS to prevent man-in-the-middle attacks and ensure the content hasn’t been tampered with.
- Discoverable: They can be identified as “applications” thanks to W3C manifests and service worker registration, allowing search engines to find them.
- Re-engageable: Features like push notifications enable developers to re-engage users with content, similar to native apps.
- Installable: PWAs can be installed on the user’s home screen without needing an app store.
- Linkable: They are easily shareable via URL and do not require complex installation processes.
Benefits of PWAs for Full-Stack Developers
Here are some key benefits PWAs offer for full-stack developers:
- Cross-Platform Compatibility: PWAs work across all platforms and devices, reducing the need to maintain separate codebases for web and mobile apps.
- Improved User Experience: With offline capabilities, fast loading times, and a seamless app-like experience, PWAs provide a superior user experience.
- SEO-Friendly: Unlike native apps, PWAs are discoverable by search engines, which can improve visibility and traffic.
- Cost-Effective Development: Developing a PWA is often cheaper and faster than building and maintaining separate native apps for different platforms.
- Lower User Acquisition Costs: Users can install PWAs directly from their browsers, bypassing the need for app stores and reducing friction in the user acquisition process.
Building a PWA: A Step-by-Step Guide
The steps for building a PWA are described across the following sections as would be covered in an inclusive java full stack developer course.
1. Create a Responsive Web Application
- Ensure your web application is responsive and works well on different devices and screen sizes.
- Use CSS frameworks like Bootstrap or Tailwind CSS to achieve responsiveness easily.
2. Use HTTPS
- Serve your application over HTTPS to ensure security. This is a requirement for many PWA features, including service workers.
3. Add a Web App Manifest
- Create a manifest.json file that provides metadata about your app, such as its name, icons, theme color, and display settings.
Example:
json
Copy code
{
“name”: “My PWA”,
“short_name”: “PWA”,
“start_url”: “/index.html”,
“display”: “standalone”,
“background_color”: “#ffffff”,
“theme_color”: “#000000”,
“icons”: [
{
“src”: “/images/icon-192×192.png”,
“type”: “image/png”,
“sizes”: “192×192”
},
{
“src”: “/images/icon-512×512.png”,
“type”: “image/png”,
“sizes”: “512×512”
}
]
}
- Link this manifest in your HTML file:
html
Copy code
<link rel=”manifest” href=”/manifest.json”>
4. Implement Service Workers
- Service workers are at the core of a PWA’s offline capabilities. They are background scripts that intercept network requests, manage caching, and enable push notifications.
- Register a service worker in your app’s main JavaScript file:
javascript
Copy code
if (‘serviceWorker’ in navigator) {
navigator.serviceWorker.register(‘/service-worker.js’)
.then(function(registration) {
console.log(‘Service Worker registered with scope:’, registration.scope);
})
.catch(function(error) {
console.log(‘Service Worker registration failed:’, error);
});
}
Example of a basic service worker:
javascript
Copy code
const CACHE_NAME = ‘my-pwa-cache-v1’;
const urlsToCache = [
‘/’,
‘/styles/main.css’,
‘/script/main.js’
];
self.addEventListener(‘install’, function(event) {
event.waitUntil(
caches.open(CACHE_NAME)
.then(function(cache) {
return cache.addAll(urlsToCache);
})
);
});
self.addEventListener(‘fetch’, function(event) {
event.respondWith(
caches.match(event.request)
.then(function(response) {
return response || fetch(event.request);
})
);
});
5. Ensure App-Like Navigation
- Configure the web app manifest and service worker to ensure your PWA loads quickly and feels like a native app. This includes setting the display property to standalone and caching key resources.
6. Test Offline Capabilities
- Use tools like Chrome DevTools to simulate offline scenarios and ensure your app works as expected without an internet connection.
- Make sure all essential parts of the app are accessible offline.
7. Add Push Notifications
- Implement push notifications to keep users engaged. This requires integrating with a service like Firebase Cloud Messaging (FCM).
Example:
javascript
Copy code
Notification.requestPermission(function(status) {
console.log(‘Notification permission status:’, status);
});
function displayNotification() {
if (Notification.permission == ‘granted’) {
navigator.serviceWorker.getRegistration().then(function(reg) {
reg.showNotification(‘Hello World!’);
});
}
}
8. Optimise Performance
- Ensure fast loading times by optimising assets, minimising JavaScript and CSS, and leveraging service workers for caching.
- Use tools like Google Lighthouse to audit your PWA for performance, accessibility, and best practices.
Best Practices for PWAs
Most full stack developer classes end by providing learners with some best practices and tips that will be greatly useful to them in their professional roles. Some of the most useful ones are described here.
- Optimise for Performance: Focus on fast loading times, both on initial load and subsequent navigations. Minimise JavaScript, CSS, and other assets, and use lazy loading where appropriate.
- Provide an Install Prompt: Use the before-installprompt event to provide users with a custom install prompt, encouraging them to add the PWA to their home screen.
- Use Reliable Caching Strategies: Choose appropriate caching strategies based on the nature of your app (for example, cache-first, network-first, stale-while-revalidate) to ensure a balance between performance and freshness.
- Monitor and Update: Regularly monitor your PWA’s performance and update the service worker to handle changes in assets or content. Ensure users get the latest version of the app without compromising their experience.
- Accessibility: Ensure your PWA is accessible to all users, including those with disabilities. Follow accessibility best practices and guidelines to make your app usable for everyone.
Conclusion
Progressive Web Apps offer a powerful way for full-stack developers to create applications that provide a native-like experience on the web. By leveraging the key features of PWAs—such as offline functionality, push notifications, and responsive design—you can build highly engaging and performant web applications that work across all devices and platforms. Many developers are enrolling in a Java full stack developer course as they have realized that understanding and implementing PWAs is essential for delivering superior user experiences and reaching a broader audience.
Business Name: ExcelR – Full Stack Developer And Business Analyst Course in Bangalore
Address: 10, 3rd floor, Safeway Plaza, 27th Main Rd, Old Madiwala, Jay Bheema Nagar, 1st Stage, BTM 1st Stage, Bengaluru, Karnataka 560068
Phone: 7353006061
Business Email: [email protected]